Mailchimp integration
This tutorial will tell you how to integrate Mailchimp webhooks with Messente's Omnichannel API to send out SMS, Whatsapp and other messages.
Step 1 - Set up webhook
FIrst you will need a script running on your web server that handles callbacks from Mailchimp that you will set up in next step.
Here is an example of a PHP script that illustrates sending out confirmations via SMS to an user who has just signed up or unsubscribed:
<?php
// Log the request
syslog(LOG_DEBUG, json_encode($_POST));
// composer require messente/messente-api-php
require_once(__DIR__ . '/config.php');
require_once(__DIR__ . '/vendor/autoload.php');
use \Messente\Api\Api\OmnimessageApi;
use \Messente\Api\Configuration;
use \Messente\Api\Model\Omnimessage;
use \Messente\Api\Model\Viber;
use \Messente\Api\Model\SMS;
use \Messente\Api\Model\WhatsApp;
use \Messente\Api\Model\WhatsAppText;
// Configure HTTP basic authorization: basicAuth
$config = Configuration::getDefaultConfiguration()
->setUsername(MESSENTE_API_USERNAME)
->setPassword(MESSENTE_API_PASSWORD);
if (empty($_POST)) {
die('NO DATA');
}
$contact = $_POST['data']['merges'];
$apiInstance = new OmnimessageApi(new GuzzleHttp\Client(), $config);
$omnimessage = new Omnimessage([
"to" => "+".preg_replace('/\D/', '', $contact['PHONE'])
]);
if ($_POST['type'] == "unsubscribe") {
$text = "We're sad to see you leave, ".$contact['FNAME'];
} elseif ($_POST['type'] == "subscribe") {
$text = "I'm glad to see you joined with our mailing list, ".$contact['FNAME'];
} else {
die('SKIP ACTION');
}
$sms = new SMS([
"text" => $text,
"sender" => "Test"
]);
$omnimessage->setMessages([$sms]);
try {
$result = $apiInstance->sendOmnimessage($omnimessage);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling OmnimessageApi->sendOmnimessage: ', $e->getMessage(), PHP_EOL;
}
die('NOTIFIED');
Step 2 - Add webhook to an existing audience
In the first step you will need to assign a trigger to an audience under Settings > Webhooks.
Here you can pick which action types trigger the Callback URL - in current example we have selected only "subscribe" and "unsubscribe" types made by the subscriber themselves.
The Callback URL must be set to the endpoint where the script has been configured in Step 1.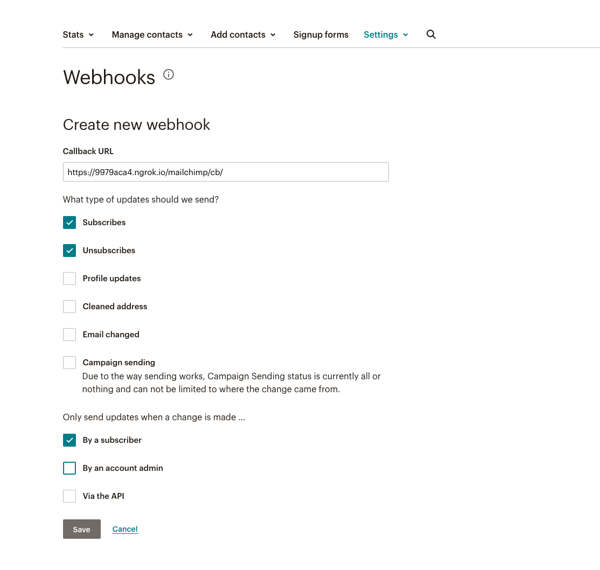
Step 3 - Set up Signup Form
Last step is to add a signup form for the Audience so that you can start getting new contacts to Mailchimp.
Please refer to "Add a Signup Form to your Website" section on how to set up Signup form.